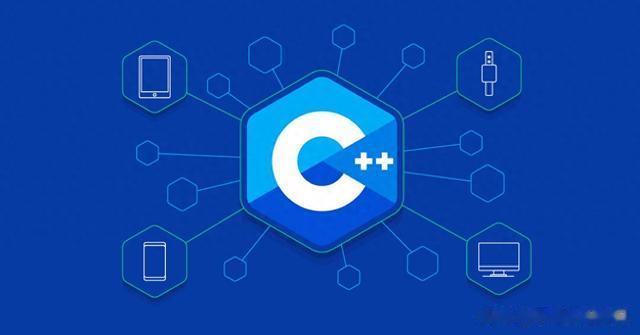
在数据存储和传输过程中,压缩技术扮演着至关重要的角色。通过压缩,我们可以有效地减少数据的大小,从而节省存储空间并加快传输速度。在众多压缩库中,Minizip以其出色的性能和广泛的应用而备受瞩目。本文将详细介绍Minizip这一强大的压缩开源库,并通过C++代码示例展示其使用方法。
一、Minizip库概述Minizip是一个基于zlib的压缩库,它提供了丰富的API来支持多种压缩格式,包括ZIP、GZIP等。Minizip以其简洁的接口、高效的压缩算法和广泛的兼容性而受到开发者的青睐。无论是在Windows、Linux还是Mac OS等操作系统上,Minizip都能表现出色。
Minizip的主要特点包括:
支持多种压缩格式:Minizip能够处理ZIP、GZIP等多种压缩格式,满足不同的压缩需求。高效的压缩算法:基于zlib的压缩算法,Minizip能够实现高效的压缩效果,减少数据的大小。简洁的API接口:Minizip提供了简洁易用的API接口,使得开发者能够轻松地在项目中集成和使用。广泛的兼容性:Minizip能够在多种操作系统和平台上运行,具有良好的兼容性。二、Minizip库的主要功能Minizip库提供了丰富的功能,包括压缩、解压、文件遍历等。以下是Minizip库的一些主要功能:
压缩功能:Minizip能够将一个或多个文件压缩成ZIP或GZIP格式,减少文件的大小。解压功能:Minizip能够解压ZIP或GZIP格式的文件,还原出原始的文件内容。文件遍历功能:Minizip提供了遍历ZIP文件内所有条目的功能,方便开发者对ZIP文件进行操作。错误处理功能:Minizip提供了完善的错误处理机制,能够捕获并处理压缩或解压过程中出现的错误。三、Minizip库的C++代码示例为了更好地展示Minizip库的使用方法,以下是一个C++代码示例,演示了如何使用Minizip库进行文件的压缩和解压。
首先,确保你已经安装了Minizip库。在Ubuntu系统上,你可以通过以下命令安装:
sudo apt-get install libminizip-dev接下来,我们将编写一个C++程序,该程序将使用Minizip库来压缩和解压文件。
#include #include #include #include // 压缩文件函数bool compressFile(const std::string& sourceFile, const std::string& zipFile) { zipFile_t zipfile = zipOpen(zipFile.c_str(), APPEND_STATUS_CREATE); if (!zipfile) { std::cerr << "Failed to create zip file: " << zipFile << std::endl; return false; } zip_fileinfo zi; memset(&zi, 0, sizeof(zip_fileinfo)); if (zipOpenNewFileInZip(zipfile, sourceFile.c_str(), &zi, NULL, 0, NULL, 0, NULL, Z_DEFLATED, Z_DEFAULT_COMPRESSION) != ZIP_OK) { std::cerr << "Failed to open file in zip: " << sourceFile << std::endl; zipClose(zipfile, NULL); return false; } FILE* fin = fopen(sourceFile.c_str(), "rb"); if (!fin) { std::cerr << "Failed to open source file: " << sourceFile << std::endl; zipCloseFileInZip(zipfile); zipClose(zipfile, NULL); return false; } char buffer[4096]; int len; while ((len = fread(buffer, 1, sizeof(buffer), fin)) > 0) { if (zipWriteInFileInZip(zipfile, buffer, len) != ZIP_OK) { std::cerr << "Failed to write to zip file" << std::endl; fclose(fin); zipCloseFileInZip(zipfile); zipClose(zipfile, NULL); return false; } } fclose(fin); zipCloseFileInZip(zipfile); zipClose(zipfile, NULL); return true;}// 解压文件函数bool extractFile(const std::string& zipFile, const std::string& extractDir) { unzFile uf = unzOpen(zipFile.c_str()); if (!uf) { std::cerr << "Failed to open zip file: " << zipFile << std::endl; return false; } unz_global_info gi; if (unzGetGlobalInfo(uf, &gi) != UNZ_OK) { std::cerr << "Failed to get global info from zip file" << std::endl; unzClose(uf); return false; } char filename[256]; for (uLong i = 0; i < gi.number_entry; ++i) { if (unzGetCurrentFileInfo(uf, &filename[0], sizeof(filename), NULL, 0, NULL, 0, NULL) != UNZ_OK) { std::cerr << "Failed to get current file info" << std::endl; unzClose(uf); return false; } const std::string fullPath = extractDir + "/" + filename; if (unzOpenCurrentFile(uf) != UNZ_OK) { std::cerr << "Failed to open current file in zip" << std::endl; unzClose(uf); return false; } FILE* fout = fopen(fullPath.c_str(), "wb"); if (!fout) { std::cerr << "Failed to create extract file: " << fullPath << std::endl; unzCloseCurrentFile(uf); unzClose(uf); return false; } char buffer[4096]; int len; while ((len = unzReadCurrentFile(uf, buffer, sizeof(buffer))) > 0) { if (fwrite(buffer, len, 1, fout) != 1) { std::cerr << "Failed to write to extract file" << std::endl; fclose(fout); unzCloseCurrentFile(uf); unzClose(uf); return false; } } fclose(fout); unzCloseCurrentFile(uf); if ((i + 1) < gi.number_entry) { if (unzGoToNextFile(uf) != UNZ_OK) { std::cerr << "Failed to go to next file in zip" << std::endl; unzClose(uf); return false; } } } unzClose(uf); return true;}int main() { std::string sourceFile = "example.txt"; std::string zipFile = "example.zip"; std::string extractDir = "extracted"; // 压缩文件 if (compressFile(sourceFile, zipFile)) { std::cout << "File compressed successfully: " << zipFile << std::endl; } else { std::cerr << "Failed to compress file" << std::endl; return 1; } // 解压文件 if (extractFile(zipFile, extractDir)) { std::cout << "File extracted successfully to: " << extractDir << std::endl; } else { std::cerr << "Failed to extract file" << std::endl; return 1; } return 0;}在这个示例中,我们定义了两个函数:compressFile用于压缩文件,extractFile用于解压文件。main函数中,我们首先压缩了一个名为example.txt的文件,然后将其解压到extracted目录下。
四、Minizip库的使用注意事项在使用Minizip库时,需要注意以下几点:
确保安装了Minizip库:在使用Minizip库之前,需要确保已经正确安装了该库。可以通过包管理器或从源代码编译安装。包含必要的头文件:在代码中,需要包含Minizip库的头文件,以便使用其提供的API。处理错误和异常情况:在使用Minizip库进行压缩或解压时,需要妥善处理可能出现的错误和异常情况,确保程序的稳定性。注意内存管理:在使用Minizip库时,需要注意内存的管理,避免内存泄漏等问题。